标签页间消息传递
- 01 Apr, 2024
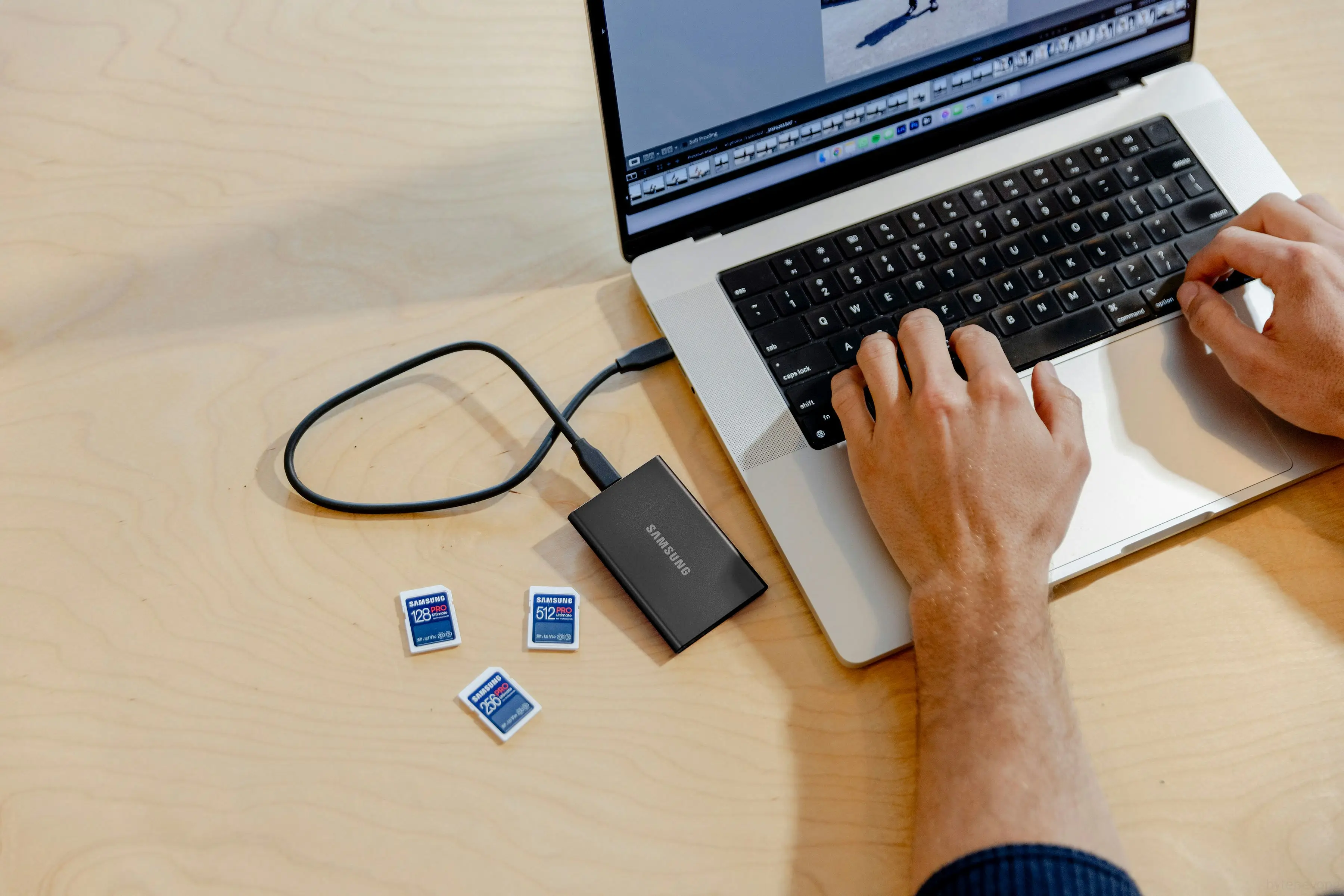
BroadcastChannel 是什么?
BroadcastChannel 是一种用于在不同的浏览器标签页之间进行通信的 API。使用 BroadcastChannel,您可以创建一个“通道”,然后在多个页面的 JavaScript 代码之间发送和接收消息。这对于需要在浏览器标签页之间同步数据的应用程序非常有用。
BroadcastChannel 的原理是什么?
BroadcastChannel API 依赖于浏览器发布和监听消息事件,从而实现了分布式事件驱动的通信模型。当一个页面向一个特定的通道发送一条消息时,所有监听该通道的页面都会收到该消息。消息传递的过程不受发送者和接收者的顺序和网络延迟的影响。
如何使用 BroadcastChannel?
使用 BroadcastChannel 的基本步骤如下:
- 创建通道对象:
const channel = new BroadcastChannel('my-channel');
- 向通道发送消息:
channel.postMessage({ message: 'hello, BroadcastChannel!' });
- 监听来自其他页面的通道消息:
channel.onmessage = event => {
console.log('Received message: ', event.data.message);
};
- 关闭通道(可选步骤):
channel.close();
如何封装 BroadcastChannel?
封装 BroadcastChannel 可以将代码组织得更好,并简化在应用程序中复用广播通道的代码。封装基本上是通过创建一个 JavaScript 类/模块,其中封装了创建、使用和关闭广播通道所需的所有逻辑。
以下是一个封装 BroadcastChannel 的示例类:
export default class BroadcastChannelHelper {
constructor(channelName) {
this.channel = undefined;
this.channelName = channelName;
}
start(callback) {
this.channel = new BroadcastChannel(this.channelName);
this.channel.onmessage = event => {
if (typeof callback === 'function') {
callback(event.data);
}
};
}
stop() {
if (this.channel) {
this.channel.close();
this.channel = undefined;
}
}
postMessage(message) {
if (this.channel) {
this.channel.postMessage(message);
}
}
}
使用技巧和注意事项
- BroadcastChannel API 不允许在同一页面的不同上下文中往同一通道发送消息,比如,如果您在同一页面中同时使用iframe,那么这些iframe不得使用同样的广播通道。
- 不同浏览器对 BroadcastChannel 的支持存在差异,如果需要跨浏览器或非常老的浏览器,则需要使用其他技术。
- 当一个页面关闭后,它所侦听的通道也会被关闭。因此,页面的未处理的消息,将丢失。
总结
BroadcastChannel 是一种非常便捷的浏览器标签页间通信 API。使用 BroadcastChannel,您可以创建一个广播通道,并安全地在多个页面之间传递消息。通过使用 BroadcastChannel API,您可以创建一个在现代浏览器之间高效运行的消息传递应用程序。